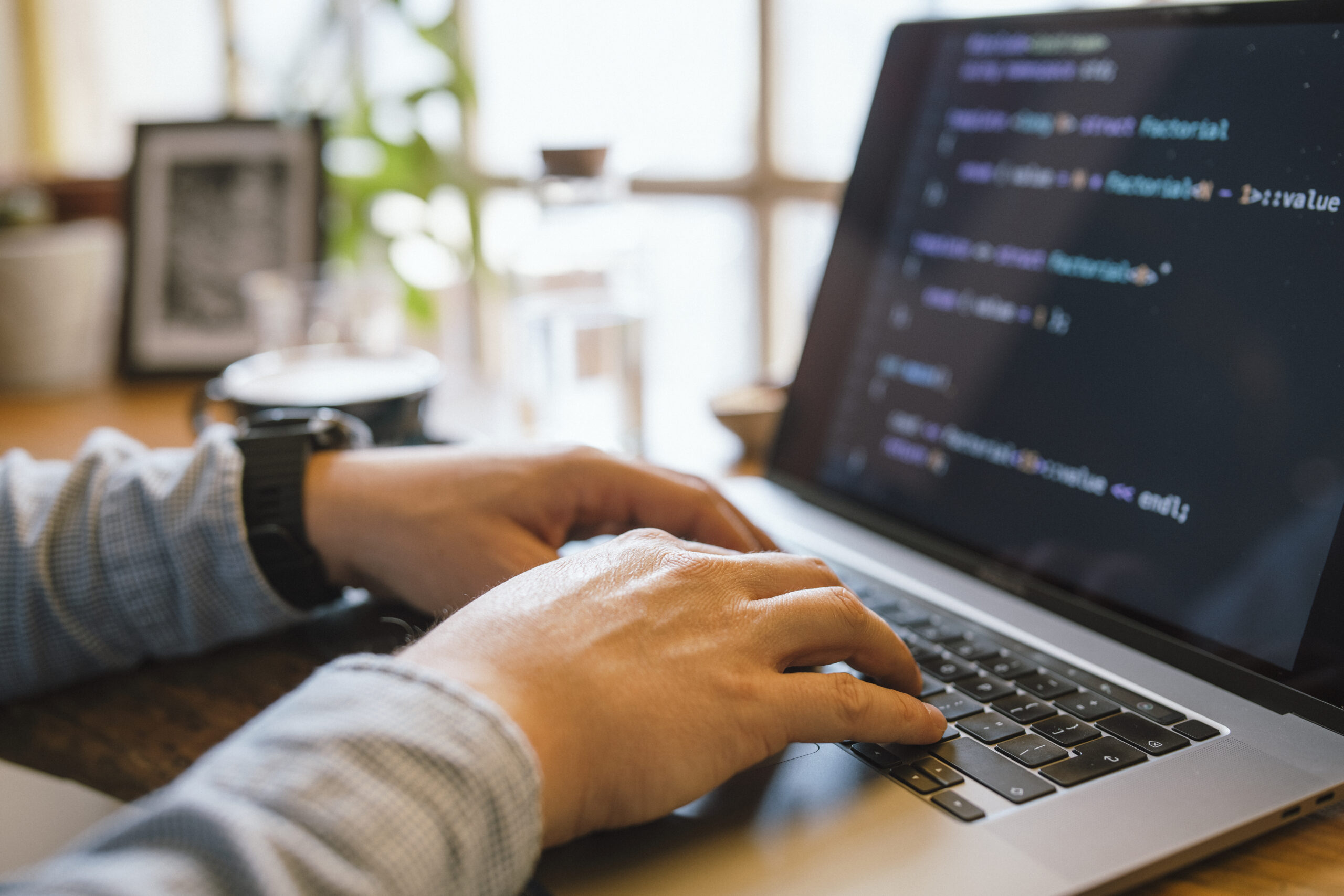
Debugging is One of the more important — nevertheless generally missed — abilities within a developer’s toolkit. It is not almost repairing broken code; it’s about knowledge how and why things go wrong, and Studying to Believe methodically to solve issues effectively. Regardless of whether you're a newbie or even a seasoned developer, sharpening your debugging expertise can conserve hours of aggravation and significantly enhance your productivity. Here are quite a few procedures that will help builders degree up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
One of many quickest ways builders can elevate their debugging capabilities is by mastering the equipment they use each day. While crafting code is a person Component of growth, realizing how you can connect with it properly in the course of execution is equally significant. Present day advancement environments come Outfitted with effective debugging abilities — but quite a few developers only scratch the area of what these equipment can do.
Take, such as, an Built-in Improvement Ecosystem (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These tools help you set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and perhaps modify code about the fly. When used effectively, they let you notice exactly how your code behaves for the duration of execution, that is priceless for monitoring down elusive bugs.
Browser developer resources, which include Chrome DevTools, are indispensable for front-conclusion developers. They enable you to inspect the DOM, monitor network requests, watch genuine-time effectiveness metrics, and debug JavaScript within the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable duties.
For backend or process-level developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of functioning processes and memory management. Finding out these instruments may have a steeper Understanding curve but pays off when debugging effectiveness challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be relaxed with Variation Command methods like Git to comprehend code historical past, come across the precise instant bugs were being introduced, and isolate problematic modifications.
In the end, mastering your equipment signifies heading outside of default options and shortcuts — it’s about producing an personal familiarity with your progress ecosystem so that when issues arise, you’re not lost in the dark. The better you understand your equipment, the more time you are able to invest solving the particular trouble rather then fumbling as a result of the procedure.
Reproduce the challenge
The most essential — and sometimes ignored — actions in effective debugging is reproducing the condition. Right before leaping to the code or creating guesses, builders will need to make a constant environment or state of affairs wherever the bug reliably appears. Without reproducibility, correcting a bug gets a sport of chance, normally resulting in wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as feasible. Check with queries like: What steps resulted in the issue? Which natural environment was it in — advancement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you have, the less complicated it gets to be to isolate the precise situations less than which the bug happens.
As you’ve collected more than enough details, try to recreate the challenge in your local setting. This may suggest inputting a similar info, simulating identical user interactions, or mimicking process states. If the issue appears intermittently, look at composing automatic tests that replicate the edge scenarios or state transitions concerned. These assessments not only aid expose the condition but additionally avert regressions Down the road.
Occasionally, The problem can be environment-certain — it would materialize only on certain working programs, browsers, or less than particular configurations. Making use of equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a action — it’s a mentality. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you might be already halfway to fixing it. With a reproducible scenario, You should use your debugging resources a lot more efficiently, examination prospective fixes securely, and talk a lot more Obviously along with your group or customers. It turns an abstract complaint right into a concrete obstacle — Which’s in which developers thrive.
Read and Have an understanding of the Mistake Messages
Mistake messages are sometimes the most precious clues a developer has when one thing goes Incorrect. Instead of seeing them as disheartening interruptions, builders need to find out to treat mistake messages as immediate communications through the program. They frequently show you just what exactly took place, in which it happened, and from time to time even why it took place — if you know how to interpret them.
Get started by looking at the message carefully As well as in complete. Many builders, especially when less than time strain, glance at the primary line and instantly start generating assumptions. But deeper from the error stack or logs may perhaps lie the real root cause. Don’t just duplicate and paste error messages into search engines like google and yahoo — go through and understand them 1st.
Break the error down into parts. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it position to a specific file and line variety? What module or function induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often comply with predictable styles, and Studying to acknowledge these can greatly quicken your debugging approach.
Some faults are vague or generic, and in All those cases, it’s vital to look at the context wherein the error occurred. Check out similar log entries, input values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial challenges and supply hints about prospective bugs.
In the long run, mistake messages are not your enemies—they’re your guides. Studying to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties a lot quicker, reduce debugging time, and become a much more effective and assured developer.
Use Logging Properly
Logging is The most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s occurring beneath the hood while not having to pause execution or phase throughout the code line by line.
An excellent logging method begins with understanding what to log and at what level. Common logging concentrations involve DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for in-depth diagnostic information and facts through progress, Data for basic occasions (like successful get started-ups), Alert for prospective problems that don’t crack the appliance, ERROR for actual challenges, and Deadly when the process can’t keep on.
Steer clear of flooding your logs with too much or irrelevant facts. A lot of logging can obscure essential messages and decelerate your program. Concentrate on vital functions, state variations, input/output values, and critical final decision points in the code.
Format your log messages Evidently and constantly. Include context, for instance timestamps, request IDs, and performance names, so it’s easier to trace difficulties in distributed devices or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even much easier to parse and filter logs programmatically.
Throughout debugging, logs Enable you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting the program. They’re Primarily useful in output environments in which stepping by code isn’t feasible.
Also, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. Using a very well-thought-out logging strategy, you could reduce the time it requires to identify challenges, acquire deeper visibility into your apps, and Increase the overall maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not just a specialized undertaking—it is a method of investigation. To successfully recognize and deal with bugs, builders must method the process just like a detective fixing a thriller. This way of thinking allows stop working complex problems into manageable elements and comply with clues logically to uncover the basis bring about.
Start out by accumulating proof. Think about the indications of the problem: mistake messages, incorrect output, or performance issues. Just like a detective surveys a crime scene, collect just as much applicable information as you are able to without the need of leaping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent photo of what’s occurring.
Upcoming, sort hypotheses. Check with by yourself: What may be triggering this conduct? Have any modifications lately been made into the codebase? Has this difficulty transpired ahead of below equivalent situations? The goal should be to slim down opportunities and recognize possible culprits.
Then, test your theories systematically. Endeavor to recreate the challenge within a managed environment. For those who suspect a certain perform or ingredient, isolate it and confirm if the issue persists. Similar to a detective conducting interviews, request your code questions and Permit the outcomes guide you closer to the truth.
Fork out close notice to modest particulars. Bugs normally conceal in the minimum expected spots—like a missing semicolon, an off-by-one error, or a race issue. Be thorough and individual, resisting the urge to patch here the issue with no fully comprehension it. Temporary fixes may possibly hide the true challenge, only for it to resurface later on.
Last of all, maintain notes on That which you tried and realized. Equally as detectives log their investigations, documenting your debugging process can preserve time for upcoming problems and support Many others realize your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic complications methodically, and turn out to be simpler at uncovering concealed challenges in complex methods.
Publish Assessments
Crafting tests is one of the most effective strategies to transform your debugging competencies and overall improvement efficiency. Exams not merely support capture bugs early and also function a security Web that offers you assurance when earning changes to your codebase. A nicely-tested application is easier to debug because it allows you to pinpoint exactly where and when a problem occurs.
Get started with device assessments, which center on particular person features or modules. These modest, isolated exams can swiftly reveal whether or not a specific bit of logic is Doing the job as predicted. Every time a examination fails, you right away know exactly where to appear, substantially decreasing the time used debugging. Device exams are Particularly useful for catching regression bugs—difficulties that reappear immediately after Formerly becoming preset.
Upcoming, integrate integration tests and close-to-conclude exams into your workflow. These help be sure that a variety of elements of your software operate with each other effortlessly. They’re notably helpful for catching bugs that manifest in intricate methods with various elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Composing tests also forces you to definitely think critically regarding your code. To check a attribute properly, you may need to understand its inputs, predicted outputs, and edge cases. This amount of understanding In a natural way leads to higher code composition and fewer bugs.
When debugging a problem, crafting a failing check that reproduces the bug might be a robust first step. When the exam fails regularly, you may focus on repairing the bug and enjoy your exam pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In short, creating exams turns debugging from the disheartening guessing game into a structured and predictable method—serving to you capture more bugs, quicker and a lot more reliably.
Acquire Breaks
When debugging a tough difficulty, it’s simple to become immersed in the trouble—watching your display screen for hrs, hoping Alternative after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks assists you reset your thoughts, lessen stress, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for too long, cognitive fatigue sets in. You might get started overlooking noticeable faults or misreading code that you choose to wrote just several hours previously. On this state, your brain becomes fewer successful at challenge-fixing. A short wander, a espresso split, or perhaps switching to a distinct activity for 10–quarter-hour can refresh your emphasis. Several developers report finding the foundation of a difficulty after they've taken the perfect time to disconnect, allowing their subconscious perform within the history.
Breaks also enable avoid burnout, Particularly during extended debugging periods. Sitting down before a screen, mentally trapped, is not just unproductive but also draining. Stepping absent enables you to return with renewed Electrical power and also a clearer attitude. You might quickly discover a lacking semicolon, a logic flaw, or even a misplaced variable that eluded you right before.
In case you’re stuck, a superb rule of thumb is usually to set a timer—debug actively for 45–sixty minutes, then take a five–ten minute crack. Use that time to maneuver around, stretch, or do a little something unrelated to code. It might experience counterintuitive, Specifically less than tight deadlines, but it surely actually brings about faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of resolving it.
Discover From Every single Bug
Each individual bug you encounter is much more than simply A brief setback—It is really an opportunity to develop being a developer. Irrespective of whether it’s a syntax mistake, a logic flaw, or perhaps a deep architectural concern, each can train you a little something valuable in the event you take some time to mirror and assess what went Erroneous.
Get started by inquiring yourself a few important queries after the bug is settled: What induced it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code evaluations, or logging? The solutions usually reveal blind spots inside your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs can even be an outstanding practice. Hold a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you can proactively steer clear of.
In team environments, sharing Anything you've figured out from a bug together with your friends might be Specifically powerful. Irrespective of whether it’s by way of a Slack message, a brief publish-up, or a quick know-how-sharing session, supporting Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a much better Finding out culture.
More importantly, viewing bugs as classes shifts your state of mind from irritation to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as vital elements of your progress journey. In any case, a lot of the greatest builders usually are not those who create great code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a different layer for your ability established. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, keep in mind: debugging isn’t a chore — it’s a chance to become superior at Anything you do.